
Are you looking to create a web application that allows you to manage data? Then, you need a React JS CRUD Application (Create, Read, Update, Delete) application. A CRUD application enables you to perform basic database operations like creating new records, reading existing ones, updating them, and deleting them.
We will implement these below functionality .
how to create React JS CRUD Application
- how to post data using react js
- how to view data after posting the data
- how to delete data using checkboxes using react js
- how to edit data using checkboxes using react js
In this post, we’ll guide you through the process of creating a CRUD application using a popular web development framework called React. Here’s what you’ll need:
- Basic knowledge of React and JavaScript
- A code editor like Visual Studio Code
- A local development environment set up on your computer
Step 1: Set up your environment
Before you begin, you need to set up your development environment. You’ll need to install Node.js and NPM (Node Package Manager) to manage your dependencies. You can download them from the official Node.js website. Once installed, you can create a new React app using the create-react-app command. Run the following command in your terminal:
npx create-react-app my-app
cd my-app
npm start

Lets Design Navbar first using bootstrap classes so first install bootstrap by installing bootstrap classes.
npm i bootstrap@5.3.0-alpha3
Nav.js
Here is the code for the navbar just copy and paste into the Nav.js file
import React from "react";
import Container from "react-bootstrap/Container";
import Navbar from "react-bootstrap/Navbar";
import Nav from "react-bootstrap/Nav";
import "./index.css";
const Nave = () => {
return (
<>
<section>
<Navbar bg="primary" expand="lg">
<Container className="p-3">
<Navbar.Brand className="text-white" href="#home">
ziontutorial
</Navbar.Brand>
<Navbar.Toggle aria-controls="basic-navbar-nav" />
<Navbar.Collapse className="justify-content-end">
<Nav className="me-auto justify-content-end">
<Nav.Link className="text-white " href="/">
Home
</Nav.Link>
<Nav.Link className="text-white" href="/view">
Employee
</Nav.Link>
</Nav>
</Navbar.Collapse>
</Container>
</Navbar>
</section>
</>
);
};
export default Nave;
After that also install json-server for handling. Here is the npm package you have to install it . If you want to explore more about this package just link on the below link.

After installation of this package just make sure you have to put this in a package.json file so that you can use it for backend server.

These two lines put in your package.json file. Also, download concurrently package for run your backed and frontend at the same time .
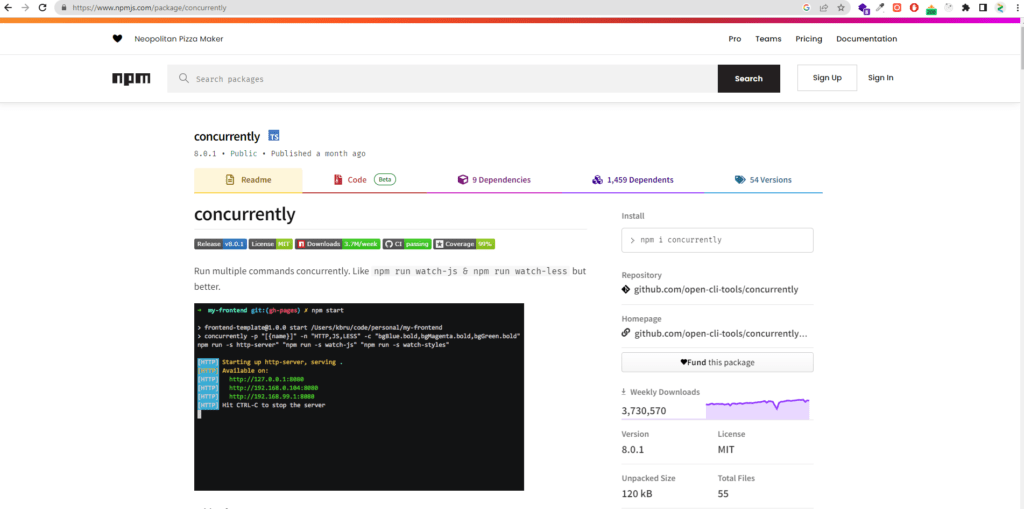
"json-server": "json-server --watch db.json --port 3003",
"start:dev": "concurrently \"npm start\" \"npm run json-server\"",
That’s it , You have to put in your package .json file .
after that you have to make a db.json file for data part . suppose if you want to store data then you can use this db.json file this will come with json server .


App.js
In app.js we are implementing our routes . So that we can jump from one link to another URL through routes package which we have to install.
The react-router-dom package contains bindings for using React Router in web applications.
npm i react-router-dom
This package help you to call for routing.
App.js Code
import Add from "./Add";
import { BrowserRouter, Routes, Route } from "react-router-dom";
import Nave from "./Nav";
import CheckboxTable from "./CheckboxTable";
import Deletemultiplerow from "./Deletemultiplerow";
const App = () => {
return (
<BrowserRouter>z
<div className="App">
<Nave />
<>
<Routes>
<Route path="/" element={<Add />} />
<Route path="/view" element={<CheckboxTable />} />
</Routes>
</>
</div>
</BrowserRouter>
);
};
export default App;
Add.js Code
Here in add.js for posting the data we have to write the code for it you can quickly. Follow the steps to post the data
import React, { useState } from "react";
import "./App.css";
import axios from "axios";
function App() {
const [id, idchange] = useState("");
const [username, usernamechange] = useState("");
const [email, emailchange] = useState("");
const [address, addresschange] = useState("");
const handlesubmit = (e) => {
e.preventDefault();
// console.log({ id, username, email, address });
const empdata = { id, username, email, address };
axios
.post("http://localhost:3003/employee", empdata)
.then((res) => {
console.log("res", res);
alert("saved successfully");
// window.location.reload();
})
.catch((err) => {
alert("some error is comiing ");
});
};
return (
<>
<section className="form-section mt-3">
<h1 className="heading">React Js CRUD Operation Data 😎</h1>
<form autoComplete="false" onSubmit={handlesubmit}>
<div className="input-block">
<label className="label">
ID <span className="requiredLabel">*</span>
</label>
<input
className="input"
type="text"
name="id"
value={id}
onChange={(e) => idchange(e.target.value)}
disabled="disabled"
placeholder="id"
tabIndex={-1}
required
/>
</div>
<div className="input-block">
<label className="label">
Username <span className="requiredLabel">*</span>
</label>
<input
className="input"
type="text"
name="username"
value={username}
onChange={(e) => usernamechange(e.target.value)}
placeholder="username"
tabIndex={-1}
required
/>
</div>
<div className="input-block">
<label className="label">
Email <span className="requiredLabel">*</span>
</label>
<input
className="input"
type="email"
name="email"
value={email}
onChange={(e) => emailchange(e.target.value)}
placeholder="ziontutorialofficial@gmail.com"
tabIndex={-1}
required
/>
</div>
<div className="input-block">
<label className="label">
Address <span className="requiredLabel">*</span>
</label>
<input
className="input"
type="text"
value={address}
onChange={(e) => addresschange(e.target.value)}
name="address"
tabIndex={-1}
required
/>
</div>
<button tabIndex={-1} className="submit-button">
Submit
</button>
</form>
</section>
</>
);
}
export default App;
Employe.js Code
Here Employe.js for editing the data and deleting the data functionality is there. we have to write the code for it you can quickly follow the steps to edit and delete your data.
import React, { useState, useEffect } from "react";
import axios from "axios";
import { Table, Button, Modal, Form } from "react-bootstrap";
function CheckboxTable() {
const [items, setItems] = useState([]);
const [selectedItems, setSelectedItems] = useState([]);
const [showModal, setShowModal] = useState(false);
const [editedItem, setEditedItem] = useState(null);
const [showDeleteModal, setShowDeleteModal] = useState(false);
useEffect(() => {
// Fetch data from API endpoint
axios
.get("http://localhost:3003/employee")
.then((response) => setItems(response.data))
.catch((error) => console.error(error));
}, []);
const handleCheckboxChange = (e, item) => {
if (e.target.checked) {
setSelectedItems([...selectedItems, item]);
} else {
setSelectedItems(
selectedItems.filter((selectedItem) => selectedItem.id !== item.id)
);
}
};
const handleEdit = () => {
// Open modal to edit selected item
console.log("Opening edit modal...");
setShowModal(true);
setEditedItem(selectedItems[0]);
};
const handleSave = () => {
// Update item and close modal
axios
.put(`http://localhost:3003/employee/${editedItem.id}`, editedItem)
.then((response) => {
setItems(
items.map((item) =>
item.id === editedItem.id ? response.data : item
)
);
setShowModal(false);
setEditedItem(null);
})
.catch((error) => console.error(error));
};
const handleClose = () => {
// Close modal and reset edited item
setShowModal(false);
setEditedItem(null);
};
const handleDelete = () => {
setShowDeleteModal(true);
};
const handleDeleteConfirm = () => {
// Delete selected items and close modal
const itemIds = selectedItems.map((item) => item.id);
axios
.delete(`http://localhost:3003/employee/${itemIds.join(",")}`)
.then(() => {
setItems(items.filter((item) => !itemIds.includes(item.id)));
setSelectedItems([]);
setShowDeleteModal(false);
})
.catch((error) => console.error(error));
};
const handleChange = (e) => {
// Update edited item with form data
setEditedItem({ ...editedItem, [e.target.name]: e.target.value });
};
return (
<section className="container">
<h1 className="heading">React Js CRUD Operation Data 😎</h1>
<div className="button_wrapper">
<Button
className="btn1"
onClick={handleEdit}
disabled={selectedItems.length !== 1}
>
Edit
</Button>
<Button
className="btn-2 ml-5"
onClick={handleDelete}
disabled={selectedItems.length === 0}
>
Delete
</Button>
</div>
<Table striped bordered hover className="section_wrapper">
<thead>
<tr>
<th></th>
<th>id</th>
<th>username</th>
<th>email</th>
<th>address</th>
</tr>
</thead>
<tbody>
{items.map((item) => (
<tr key={item.id}>
<td>
<Form.Check
type="checkbox"
onChange={(e) => handleCheckboxChange(e, item)}
checked={selectedItems.some(
(selectedItem) => selectedItem.id === item.id
)}
/>
</td>
<td>{item.id}</td>
<td>{item.username}</td>
<td>{item.email}</td>
<td>{item.address}</td>
</tr>
))}
</tbody>
</Table>
<Modal show={showModal} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title>Edit Item</Modal.Title>
</Modal.Header>
<Modal.Body>
<Form>
<Form.Group controlId="formBasicUsername">
<Form.Label>Username</Form.Label>
<Form.Control
type="text"
placeholder="Enter username"
name="username"
value={editedItem?.username}
onChange={handleChange}
/>
</Form.Group>
<Form.Group controlId="formBasicEmail">
<Form.Label>Email address</Form.Label>
<Form.Control
type="email"
placeholder="Enter email"
name="email"
value={editedItem?.email}
onChange={handleChange}
/>
</Form.Group>
<Form.Group controlId="formBasicAddress">
<Form.Label>Address</Form.Label>
<Form.Control
type="text"
placeholder="Enter address"
name="address"
value={editedItem?.address}
onChange={handleChange}
/>
</Form.Group>
</Form>
</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button variant="primary" onClick={handleSave}>
Save Changes
</Button>
</Modal.Footer>
</Modal>
<Modal show={showDeleteModal} onHide={() => setShowDeleteModal(false)}>
<Modal.Header closeButton>
<Modal.Title>Delete Items</Modal.Title>
</Modal.Header>
<Modal.Body>
<p>Are you sure you want to delete the selected items?</p>
</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={() => setShowDeleteModal(false)}>
Cancel
</Button>
<Button variant="danger" onClick={handleDeleteConfirm}>
Delete
</Button>
</Modal.Footer>
</Modal>
</section>
);
}
export default CheckboxTable;
Index.css
* {
box-sizing: border-box;
padding: 0;
margin: 0;
list-style: none;
text-decoration: none;
}
html,
body {
font-family: "Roboto", sans-serif;
font-size: 10px;
background-color: #f4f4f4;
}
.heading {
text-align: center;
font-size: 2.75rem;
margin-bottom: 1em;
}
.form-section {
min-height: 95vh;
display: grid;
place-content: center;
}
.section_wrapper {
place-content: center;
padding: 10rem;
}
.input-block {
display: flex;
flex-direction: column;
align-items: flex-start;
margin: 2em 0 0.5em;
}
.label {
font-family: "Roboto";
letter-spacing: 0.25px;
font-weight: 500;
font-size: 1.35rem;
color: #000;
margin-bottom: 0.35em;
}
.requiredLabel {
color: red;
font-weight: bold;
}
.input {
padding: 1.35em 1em;
width: 350px;
background-color: #fff;
outline: none;
border: 1px solid rgb(130, 130, 130);
border-radius: 0.25em;
}
.submit-button-wrapper {
display: flex;
}
.float {
justify-content: flex-end;
}
.submit-button {
margin-top: 1.75rem;
background-color: #000;
color: #fff;
letter-spacing: 0.5px;
padding: 0.85em 2.2em;
border: none;
font-size: 1.5rem;
font-weight: 500;
border-radius: 4px;
}
.button_wrapper {
margin-bottom: 1rem;
display: flex;
}
.btn {
margin-left: 10px;
padding: 0.85em 2.2em;
border: none;
font-size: 1rem;
font-weight: 500;
}
.btn-1 {
margin-left: 10px;
padding: 0.85em 2.2em;
border: none;
font-size: 1.5rem;
font-weight: 500;
}
Index.js
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
import App from "./App";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
db.json
Copy and paste the below code in db.json for employee data structure for this you have to install json server.

{
"employee": [
]
}
Conclusion
In conclusion, creating a CRUD application is an essential skill for any web developer. It allows you to manage data and build robust web applications. In this post, we walked through the steps involved in creating a CRUD application using React. We started by setting up our development environment, defining our data model, and implementing the CRUD functions. While this tutorial only scratched the surface of what’s possible with a CRUD application, it should give you a good foundation to build upon.
Remember, the beauty of a CRUD application is its simplicity. Once you’ve mastered the basics, you can build upon them to create more complex applications. Whether you’re building a personal project or working on a team, the skills you learn from creating a CRUD application will serve you well. Happy coding!
Check out this How to Install WordPress on Localhost | Xammp. Comment if you have any dought Regarding this project please comment in the comment box. Or join Our telegram Channel mostly available on that platform you can ask any question.
Happy coding!
People are also reading:
- Crypto-currency website| Source Code Free Download
- How to Build A BMI Calculator in React JS – useState Hook & Conditionals
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers