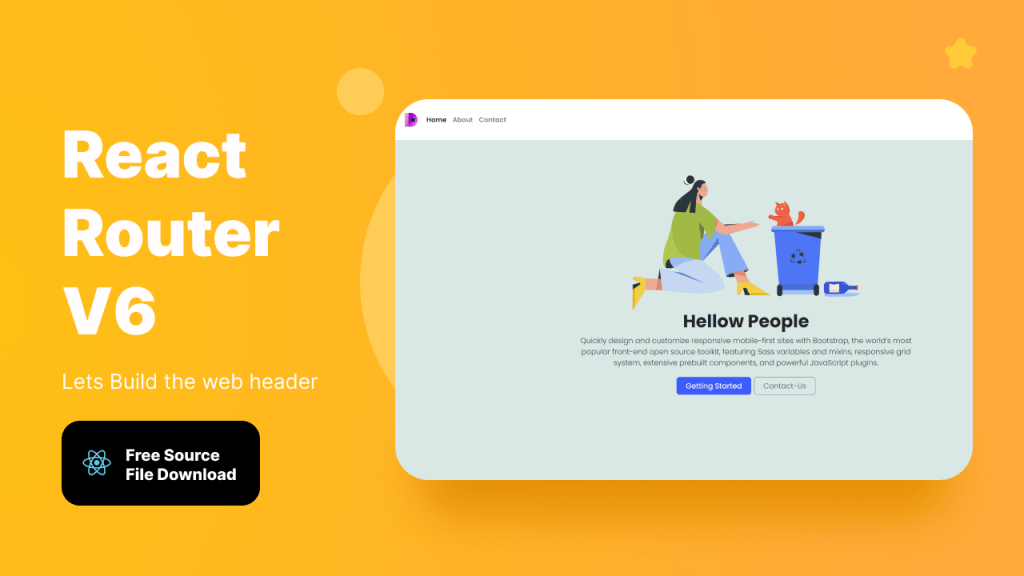
React Router is a library for routing in React js. It allows the navigation between views of various components in React Application, Which allows changing the browser URL. In this blog, we will learn about react routing or navigate through different pages by using react-router-dom. Last but not least we will make React Router v6 in React Js With a Complete Project in complete explanation.
Let’s create a simple React application to understand the flow of react-router.
In this application, the components would be the Home component, About component and the last one is Contact component so that we can jump or navigate to different components easily. You can refer to the below example of how it looks at the end of this tutorial. Or you can Follow the Youtube tutorial also.
YouTube Tutorial Video :
Let’s start making Our application by jumping to the first step
Setting Our Environment of React Application:
Create a React Application Using create-react-app. Let’s call it ziontutorial and you can use any name instead of this name. In the below example, I am using git bash you can also use cmd ( normal command prompt ) inbuilt into your system.
After the Development environment is ready. Now let’s move into the folder and open the terminal of your project and install this React Router in your Application.
Let’s Install the React Router Dom in your Application.
App.js
Here you have to import these important dependencies for performing the react routing[ import { BrowserRouter, Routes, Route } from ‘react-router-dom’; ] By importing these components you are able to perform routing easily.
import './App.css';
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import Navbar from './components/layout/Navbar';
import Home from './components/pages/Home';
import About from './components/pages/About';
import Contact from './components/pages/Contact';
function App() {
return (
<div className="App">
<BrowserRouter>
<Navbar/>
<Routes>
<Route path="/" element={<Home/>}/>
<Route path="/about" element={<About/>}/>
<Route path="/contact" element={<Contact/>}/>
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
Here is the List of React Router Components
- BrowserRouter: To keep your UI in sync with the URL, BrowserRouter is a router solution that makes use of the HTML5 history API (pushState, replaceState, and the popstate event). All of the other components are stored in the parent component.
- Routes: Routes is the new component introduced in version 6. Switch is replaced by this routes component.
- Route: it conditionally shows components and it works when routes match to the rendered components.
- Links: The Links component facilitates navigation inside the application by creating links for various paths. It functions like an anchor tag in HTML.
Now let’s make other components Like Home.js, About.js, and Contact.js so that we can jump over different components by using react-router-dom.
Navbar.js
import React from 'react'
import {Link,NavLink} from 'react-router-dom';
// import Navbar from './Navbar';
const Navbar = () => {
return (
<div className='navbar_start'>
<nav className="navbar navbar-expand-lg p-4">
<div className="container-fluid">
<NavLink className="navbar-brand" to="/">
</NavLink>
<button className="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span className="navbar-toggler-icon"></span>
</button>
<div className="collapse navbar-collapse" id="navbarNav">
<ul className="navbar-nav">
<li className="nav-item">
<NavLink className="nav-link active" aria-current="page" to="/">Home</NavLink>
</li>
<li className="nav-item">
<NavLink className="nav-link" exact to="/about">About</NavLink>
</li>
<li className="nav-item">
<NavLink className="nav-link" exact to="/contact">Contact</NavLink>
</li>
</ul>
</div>
<Link className='btn btn-outline-primary' to='/user/add'>
Add User
</Link>
</div>
</nav>
</div>
)
}
export default Navbar
Home.js
import React from 'react'
const Home = () => {
return (
<>
<div class="px-4 py-5 my-5 text-center">
<h1 class="display-5 fw-bold">Hellow People</h1>
<div class="col-lg-6 mx-auto">
<p class="lead mb-4">Quickly design and customize responsive mobile-first sites with Bootstrap, the world’s most popular front-end open source toolkit, featuring Sass variables and mixins, responsive grid system, extensive prebuilt components, and powerful JavaScript plugins.</p>
<div class="d-grid gap-2 d-sm-flex justify-content-sm-center">
<button type="button" class="btn btn-primary btn-lg px-4 gap-3">Getting Started</button>
<button type="button" class="btn btn-outline-secondary btn-lg px-4">Contact-Us</button>
</div>
</div>
</div>
</>
)
}
export default Home
About.js
import React from 'react'
const About = () => {
return (
<>
<div>
<div className="container">
<h1 className="mt-5">About Us</h1>
<p className="lead">Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.</p>
</div>
</div>
</>
)
}
export default About
Contact.js
import React from 'react'
const Contact = () => {
return (
<>
<div className='container form-signin w-100 m-auto text-center px-4 py-5 my-5 text-center'>
<div className='row'>
<main className=" ">
<form>
<h1 className="h3 mb-3 fw-normal">Please sign in</h1>
<div className="form-floating">
<input type="email" className="form-control" id="floatingInput" placeholder="name@example.com"/>
<label for="floatingInput">Email address</label>
</div>
<div className="form-floating py-5">
<input type="password" className="form-control" id="floatingPassword" placeholder="Password"/>
<label for="floatingPassword">Password</label>
</div>
<div className="checkbox mb-3">
<label>
<input type="checkbox" value="remember-me"/> Remember me
</label>
</div>
<button className="w-100 btn btn-lg btn-primary" type="submit">Sign in</button>
<p className="mt-5 mb-3 text-muted">© 2017–2022</p>
</form>
</main>
</div>
</div>
</>
)
}
export default Contact
Route: Route component will now help us to establish the link between the component’s UI and the URL. To include routes to the application, add the code given below to your app.js.
Also, match or type the same Url in navbar.js with to=”” with same as path too .
Finally Output Look like this I hope you like this blog and if you have any problem regarding this project you can connect and comment in the comment box.
Conclusion
That’s it congratulation you did it
I hope you enjoyed this little project We used react router dom to navigate to different URL . By making React Router v6 in React Js With Complete Project project you learn new things.
For more tutorials like this, you will follow our youtube channel and subscribe to our Instagram handler. I hope you like the video also which I have uploaded on youtube please like it and spread it with your friends too.
Happy Coding! ?
People are also reading:
- Crypto-currency website| Source Code Free Download
- How to Build A BMI Calculator in React JS – useState Hook & Conditionals
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers
- React JS Mini-Project #2 Creating Simple Sum Calculator | Absolute beginners useState Hook & Conditionals