This is a simple responsive BMI calculator using react js I am damn sure you will be learning a lot from this small project. We used use state hook to manage the state of the application. let’s start to Build A BMI Calculator in React JS .
Building a body mass index calculator with react js using basic class-based components and understand state and props too in a very easy way.
What Is BMI ?
Lets understand what the BmI is then we can move to the development part of this BMI calculator. it is a measurement of a person’s leanness or obesity based on their height and weight. There are many tools which helps to identify the your body bmibut in todays session we can build our own bmi calculator. Hope you are excited to do this build.
Let’s Begin making the project
Open the command prompt, go to a comfortable spot, and enter the command as follows to build the example project for this example:
npx create-react-app bmicalculator
Now, as indicated below, head to the project folder and open the folder in your fav editor. and jump to the main folder by typing
cd bmicalculator
Now just replace the placeholder content of App.js with the given below content :
let’s create this amazing BMI calculator which I have given below .
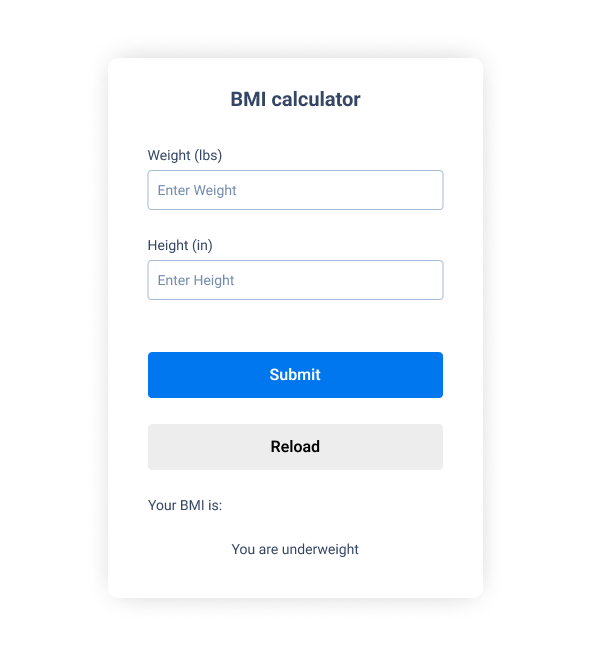
App.js
import './App.css';
import './index.css'
import React, {useState} from 'react'
function App() {
// state
const [weight, setWeight] = useState(0)
const [height, setHeight] = useState(0)
const [bmi, setBmi] = useState('')
const [message, setMessage] = useState('')
let calcBmi = (event) => {
//prevent submitting to the server
event.preventDefault()
if (weight === 0 || height === 0) {
alert('Please enter a valid weight and height')
} else {
let bmi = (weight / (height * height) * 703)
setBmi(bmi.toFixed(1))
// Logic for message
if (bmi < 25) {
setMessage('You are underweight')
} else if (bmi >= 25 && bmi < 30) {
setMessage('You are a healthy weight')
} else {
setMessage('You are overweight')
}
}
}
let reload = () => {
window.location.reload()
}
return (
<div className="app">
<div className='container'>
<h2 className='center'>BMI Calculator</h2>
<form onSubmit={calcBmi}>
<div>
<label>Weight (lbs)</label>
<input value={weight} onChange={(e) => setWeight(e.target.value)} />
</div>
<div>
<label>Height (in)</label>
<input value={height} onChange={(event) => setHeight(event.target.value)} />
</div>
<div>
<button className='btn' type='submit'>Submit</button>
<button className='btn btn-outline' onClick={reload} type='submit'>Reload</button>
</div>
</form>
<div className='center'>
<h3>Your BMI is: {bmi}</h3>
<p>{message}</p>
</div>
</div>
</div>
);
}
export default App;
In the above code, we are not using any lib for any of the logic you just create this app by making this logic to your app that’s it. It’s a very simple application of BMI and I hope you will understand the logic I will provide you with the detailed concept of this tutorial on my youtube channel just go and watch it for making this simple calculator.
Now just replace the boilerplate of index.js with the given below content :
Index.css
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
.app {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
width: 100%;
height: 100vh;
}
.container {
box-shadow: 0px 0px 12px #ccc;
border-radius: 8px;
padding: 3rem;
}
input {
width: 100%;
font-size: 1.2rem;
padding: 15px 4px;
margin: 8px 0;
border-radius: 8px;
}
.btn {
display: block;
width: 100%;
font-size: 1.2rem;
margin: 8px 0;
padding: 15px 0;
background-color: #0077EE;
color: #fff;
border: 1px solid #333;
border-radius: 8px;
cursor: pointer;
}
/* unvisited link */
.btn-outline {
background-color: #fff;
color: #A6BCDA;
}
.center {
text-align: center;
margin: 24px 0;
}
p {
margin: 10px 0;
}
.img-container {
text-align: center;
}
.img-container img {
height: 200px;
}
Index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
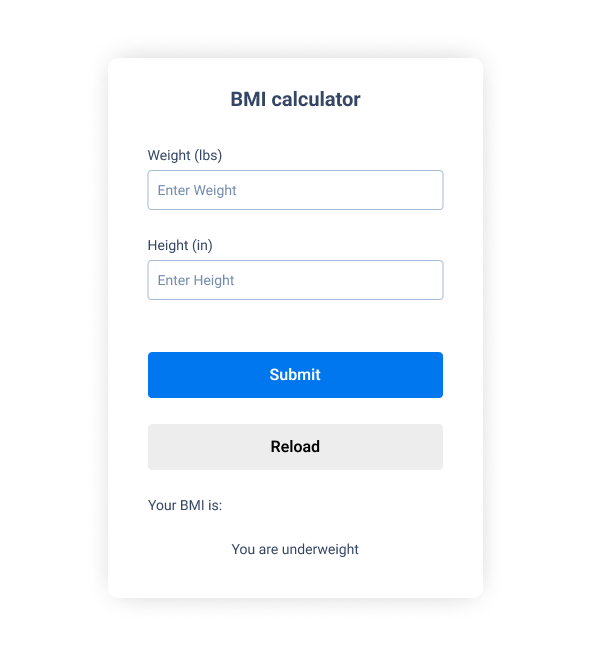
Conclusion
That’s it congratulation you did it
I hope you enjoyed this little project We used use state hook to manage the state of the application let’s start to Build A BMI Calculator in React JS.
For more tutorials like this, you will follow our youtube channel and subscribe to our Instagram handler. I hope you like the video also which I have uploaded on youtube please like it and spread it with your friends too.
Happy Coding! ?
People are also reading:
- Crypto-currency website| Source Code Free Download
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn Money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers