In this article, we will discuss how to make a dynamic currency converter using React JS. Have you ever used Google Currency converter same converter we are going to build in this article? we will be using currency exchange API to convert the currency.
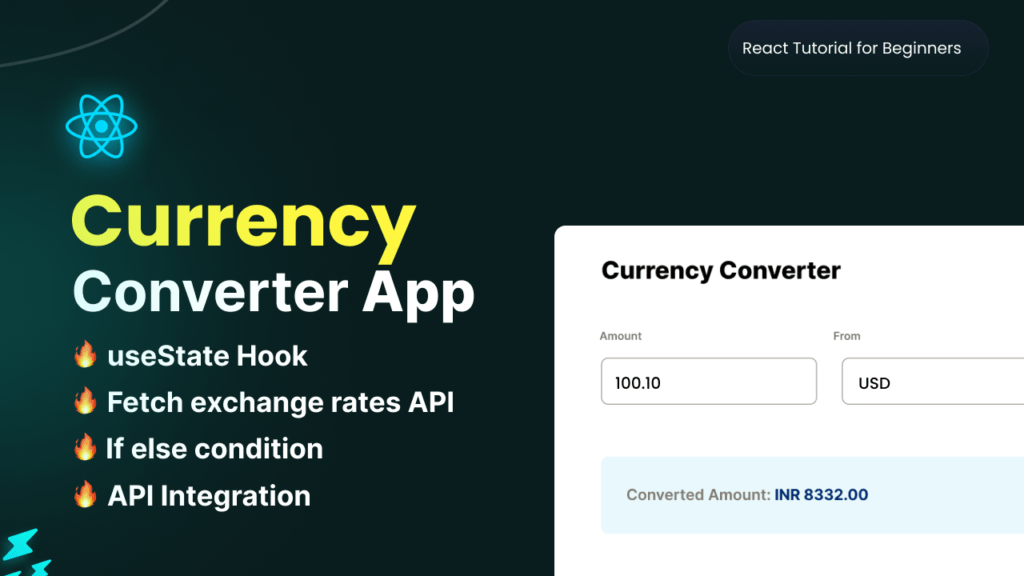
What we are going to Build ?
Fetching exchange rates
To start, we’ll set up an API to get the latest currency exchange rates. Then, we’ll set up a React app that will fetch data from the API and store it.
What is a currency converter?
According to investopedia.com it is an application that is used to easily convert currency value based on current days. By using the same concept we are going to Build a dynamic currency converter using React which will provide you with the dynamic converted values.
Let’s Begin building a currency converter using the react
Open the command prompt, go to a comfortable spot, and enter the command as follows to build the example project for this example:
Steps to create the application:
Step 1: Set up the React project using the below command in VSCode.
npx create-react-app currency_converter
Step 2: Navigate to the newly created project folder by executing the below command.
Now, as indicated below, head to the project folder and open the folder in your fav editor. and jump to the main folder by typing
cd currency_converter
Now just replace the placeholder content of App.js with the given below content :
Project Structure
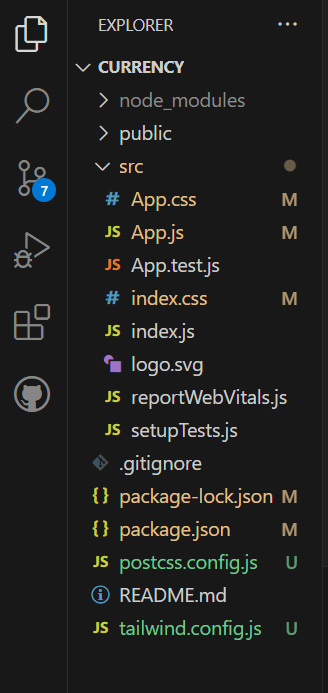
Write all the codes with the respective files so that you can achieve the required User interface and logical integration for the currency conversion.
- Index.html: This is an automatically created file in the public folder if you want to edit the title any favicon icon and any cdn if you want to put you can put them in the index.html file.
- App.js: App.js file code is the main logic of the currency converter application, where the hook (useState) is used to manage the state. We are implementing exchange rate-API also to get the currency values object for converting the amount value to the respective from and to values. You are going to use usestate fetch API if else and API integration in the app.js file.
- App.css: This is the styling file, which makes the application more attractive, we have provided some styling attributes like coloring the text, buttons, etc. Overall, all the styling is been managed through this file.
App.js
Let’s Move into the app.js file where we write all the logic that is related to the react js currency converter app.
// CurrencyConverter.js
import React, { useState, useEffect } from 'react';
import axios from 'axios';
import './App.css'
const App = () => {
const [exchangeRates, setExchangeRates] = useState({});
const [amount, setAmount] = useState(1);
const [fromCurrency, setFromCurrency] = useState('USD');
const [toCurrency, setToCurrency] = useState('INR');
const [convertedAmount, setConvertedAmount] = useState(null);
useEffect(() => {
// Fetch exchange rates from a free API (replace with your preferred API)
const apiUrl = `https://api.exchangerate-api.com/v4/latest/${fromCurrency}`;
axios.get(apiUrl)
.then(response => {
setExchangeRates(response.data.rates);
})
.catch(error => {
console.error('Error fetching exchange rates:', error);
});
}, [fromCurrency]);
useEffect(() => {
// Convert currency when 'amount', 'fromCurrency', or 'toCurrency' changes
const conversionRate = exchangeRates[toCurrency];
if (conversionRate) {
const converted = amount * conversionRate;
setConvertedAmount(converted.toFixed(2));
}
}, [amount, fromCurrency, toCurrency, exchangeRates]);
const handleChange = (e) => {
const { name, value } = e.target;
switch (name) {
case 'amount':
setAmount(value);
break;
case 'fromCurrency':
setFromCurrency(value);
break;
case 'toCurrency':
setToCurrency(value);
break;
default:
break;
}
};
return (
<div className='card' >
<h1 className='text-6xl'>Currency Converter</h1>
<div className='currency_exchnage'>
<div className="input_container" >
<label className="input_label">Amount:</label>
<input
type="number"
name="amount"
className="input_field"
value={amount}
onChange={handleChange}
/>
</div>
<div className="input_container">
<label className="input_label">From Currency:</label>
<select
name="fromCurrency"
value={fromCurrency}
onChange={handleChange}
className="input_field"
>
{Object.keys(exchangeRates).map(currency => (
<option key={currency} value={currency}>
{currency}
</option>
))}
</select>
</div>
<div className="input_container">
<label className="input_label">To Currency:</label>
<select
name="toCurrency"
value={toCurrency}
onChange={handleChange}
className="input_field"
>
{Object.keys(exchangeRates).map(currency => (
<option key={currency} value={currency}>
{currency}
</option>
))}
</select>
</div>
</div>
<div className='output'>
<h2>Converted Amount: {convertedAmount}</h2>
</div>
</div>
);
};
export default App;
App.css
Now lets write all the CSS style through which
@import url('https://fonts.googleapis.com/css2?family=Inter:wght@100;200;300;400;500;600;700;800;900');
*
{
margin: 0;
padding: 0;
box-sizing: border-box;
background-color: #F0F2F5;
font-family: "Inter", sans-serif;
}
.card
{
background-color: #ffffff;
width: 55%;
min-width: 550px;
padding: 50px;
position: absolute;
transform: translate(-50%,-50%);
top: 50%;
left: 50%;
border-radius: 8px;
}
h1{
/* padding: 16px; */
background: #fff;
font-size: 30px !important;
margin-bottom: 2rem;
font-weight: 700;
color: #333;
}
.currency_exchnage
{
display: flex;
justify-content: space-between;
background: #fff;
/* align-items: center; */
}
.input_container {
width: 30%;
height: fit-content;
position: relative;
display: flex;
flex-direction: column;
gap: 10px;
background: #fff;
}
.icon {
width: 20px;
position: absolute;
z-index: 99;
left: 12px;
bottom: 9px;
}
.input_label {
font-size: 0.95rem;
color: #8B8E98;
font-weight: 600;
background: #fff;
}
.input_field {
width: auto;
height: 60px;
background: #fff;
padding: 0 0 0 40px;
border-radius: 7px;
outline: none;
border: 1px solid #e5e5e5;
filter: drop-shadow(0px 1px 0px #efefef)
drop-shadow(0px 1px 0.5px rgba(239, 239, 239, 0.5));
transition: all 0.3s cubic-bezier(0.15, 0.83, 0.66, 1);
}
.input_field:focus {
border: 1px solid transparent;
background: #fff;
box-shadow: 0px 0px 0px 2px #242424;
background-color: transparent;
}
.output
{
background: #ffffff;
}
h2
{
width: 50%;
margin-top: 20px;
background-color: #E8F8FD;
padding: 26px;
border-radius: 10px;
}
Steps to run the application:
1. Type the following command in the terminal from your VS Code IDE.
npm start
Output:
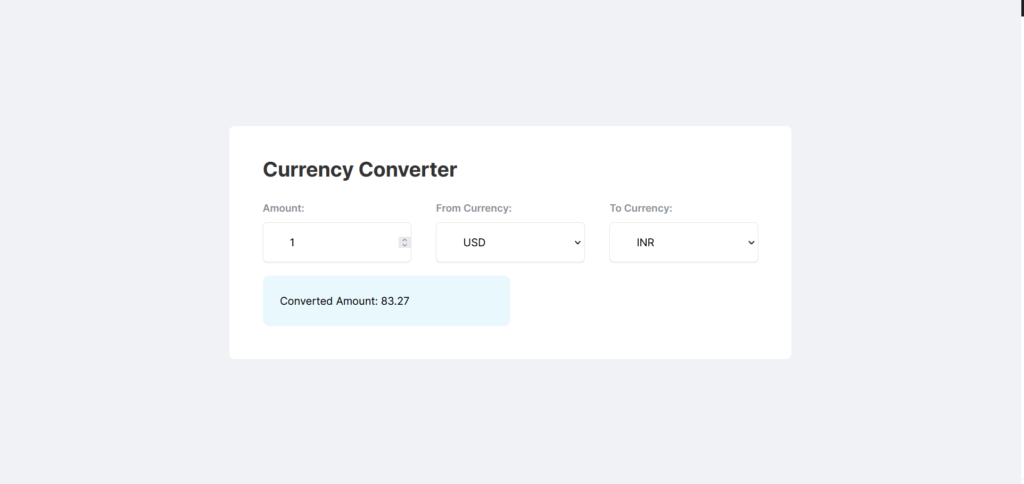
Conclusion
That’s it congratulation you did it
I hope you enjoyed this little project We used use state Hooks to manage the state of the application how to use API and how to integrate currency converter API to get the updated value according to the amount. within How to Build a Dynamic Currency Converter Using React tutorial all the beginning concepts.
For more tutorials like this, you will follow our YouTube channel and subscribe to our Instagram handler. I hope you like the video that I have uploaded on YouTube please like it and spread it with your friends too.
Happy Coding!
People are also reading:
- Crypto-currency website| Source Code Free Download
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn Money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers