This post will show you how to use React JS to make a gorgeous login form. From scratch, we translate the figma design to the react login form.
Figma Design : Link
The list of functionalities that we will build is as follows:
Create Simple Login form with React JS code
- You will learn how to design forms from Figma
- How to make this form center using react js and styling
- how to make it responsive design?
- how to use material UI to make the input box interactive.
- How to make this login form screen responsive
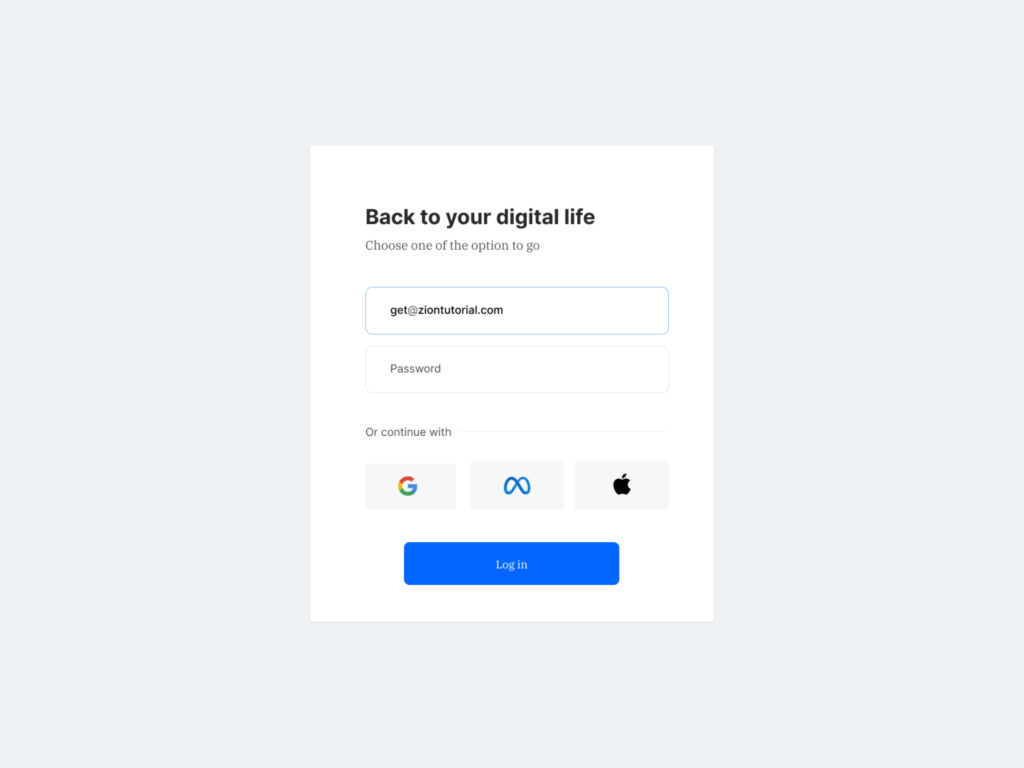
Prerequisite:
- IDE of choice (this tutorial uses VS Code, you can download it here)
- npm
- create-react-app
- sass
- flex-box
Basic Setup: To begin a new project using create-react-app, launch PowerShell or your IDE’s terminal and enter the following command:
The name of your project is “login,” but you can change it to something different like “my-first-react-website” for now.
npx create-react-app login
Enter the following command in the terminal to navigate to your react-sidebar-dropdown folder:
cd my_login
Also, install mui because we are using mui input boxes that we have to install this package. or you can visit official website for mui.
Mui installation: Link
npm install @mui/material @emotion/react @emotion/styled
Also, we are using sass for styling in this project. So we have to make it easy for styling . Install the package for sass also .
npm i sass
Folder structure
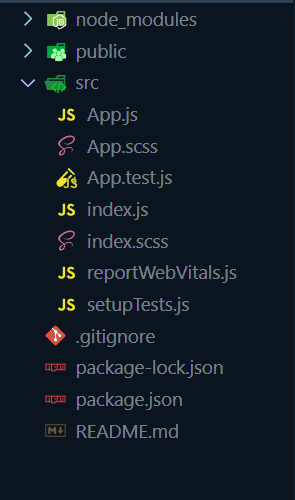
Once the packages and dependencies are finished downloading, start up your IDE and locate your project folder. Lets start Create Simple Login form with React JS code
1. App.js Code
Write this code app.js if you face any problem for designing he login page just comment in the comment box.
import "./App.scss";
import Box from "@mui/material/Box";
import TextField from "@mui/material/TextField";
function App() {
return (
<Box component="form" noValidate autoComplete="off">
<div className="App">
<div className="container">
<div className="card_area">
<h1 className="container_title">Back to your digital life</h1>
<p className="container_para">Choose one of the option to go</p>
<div className="input_card">
<TextField
className="input"
id="outlined-basic"
label="Email"
variant="outlined"
/>
<TextField
className="input"
id="outlined-basic"
label="Password"
variant="outlined"
/>
</div>
<div className="line">
<p className="line_para">Or continue with</p>
</div>
<div className="social_card">
<div className="google">
<img className="google_img" alt="" src="/1.png" />
</div>
<div className="google">
<img className="google_img" alt="" src="/2.png" />
</div>
<div className="google">
<img className="google_img" alt="" src="/3.png" />
</div>
</div>
<div className="card_button">
<button class="button-28">Log in</button>
</div>
</div>
</div>
</div>
</Box>
);
}
export default App;
Now lets move to App.sass file for styling the components.
App.scss
@import url('https://fonts.googleapis.com/css2?family=Inter:wght@100;200;300;400;500;600;700;800;900');
.container {
background-color: white;
max-width: 530px;
height: 600px;
margin: auto;
margin-top: 80px;
border-radius: 4px;
.input_card
{
margin-top: 10px;
}
.input
{
width: 100%;
margin-top: 15px;
}
.card_area
{
padding:80px;
}
.container_title
{
font-size: 30px;
font-weight: 700;
display: flex;
align-items: flex-start;
margin-bottom:-8px;
color: #292929;
}
.container_para
{
color: #545454;
font-size: 18px;
font-weight: 400;
}
.line
{
display: flex;
}
.social_card{
display: flex;
.google
{
display: flex;
align-items: center;
justify-content: center;
width: 131px;
height: 61px;
background-color: #F7F7F7;
border-radius: 4px;
margin-left: 10px;
}
}
.card_button
{
margin-top: 2rem;
.button-28 {
appearance: none;
color: #ffffff;
background-color: #0366FF;
border-radius: 15px;
border: none;
box-sizing: border-box;
color: #ffffff;
cursor: pointer;
display: inline-block;
font-family: Roobert,-apple-system,BlinkMacSystemFont,"Segoe UI",Helvetica,Arial,sans-serif,"Apple Color Emoji","Segoe UI Emoji","Segoe UI Symbol";
font-size: 16px;
font-weight: 600;
line-height: normal;
margin: 0;
min-height: 60px;
min-width: 0;
outline: none;
padding: 16px 24px;
text-align: center;
text-decoration: none;
transition: all 300ms cubic-bezier(.23, 1, 0.32, 1);
user-select: none;
-webkit-user-select: none;
touch-action: manipulation;
width: 100%;
will-change: transform;
}
.button-28:hover {
color: #fff;
background-color: #1A1A1A;
box-shadow: rgba(0, 0, 0, 0.25) 0 8px 15px;
transform: translateY(-2px);
}
.button-28:active {
box-shadow: none;
transform: translateY(0);
}
}
}
Now let’s jump to index.js file where have only written a few lines of react js. Which is only needed to run our react app. Here is the code for it.
Index.js
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.scss";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
For styling part of index.js file also follow the same step we did in app.css . Just few line of code for the index.scss code .
@import url('https://fonts.googleapis.com/css2?family=Inter:wght@100;200;300;400;500;600;700;800;900');
html,
body {
width: 100%;
height: 100%;
background-color: #eef2f5;
font-family: 'Inter', sans-serif;
display: flex;
align-items: center;
justify-content: center;
}
Conclusion
Hope you like this tutorial onCreate Simple Login form with React JS code| For more such content please visit to this website.
People are also reading:
- Crypto-currency website| Source Code Free Download
- How to Build A BMI Calculator in React JS – useState Hook & Conditionals
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers