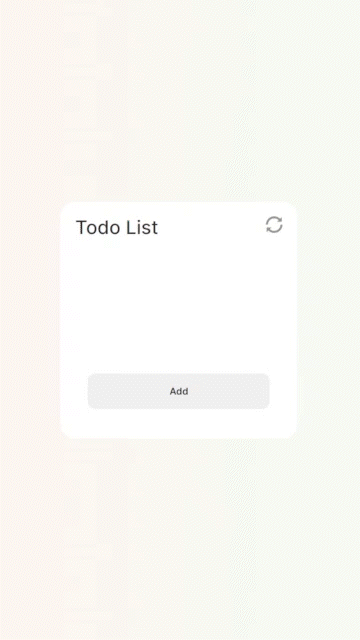
React useState is a powerful tool, so for today’s React tutorial, we will get started with building a to-do list using react js . By the end of this tutorial, you will have your first react application by using use state methods and conditional rendering.
useState
: returns a stateful value | allows adding of state to a functional component.
The list of functionalities that we will build is as follows:
- How to make this form center using react js and styling
- How to make use of useState
- How to use conditional rendering to build our logic for the to-list application.
- How to manipulate use map function to iterate the data.
- Reset the value of the input field.
- Reset the whole value.
hope you are Build A To-do list Using React JS Mini Project | useState Hook & Conditionals
Prerequisite:
- IDE of choice (this tutorial uses VS Code, you can download it here)
- npm
- create-react-app
Basic Setup: To begin a new project using create-react-app, launch PowerShell or your IDEβs terminal and enter the following command:
You can give your project name like here I m giving βmyapp,β but you can change it to something different like βmy-first-react-websiteβ for now.
npx create-react-app
Enter the following command in the terminal to navigate to your react-sidebar-dropdown folder:
cd my_login
Here is the folder structure for our application.
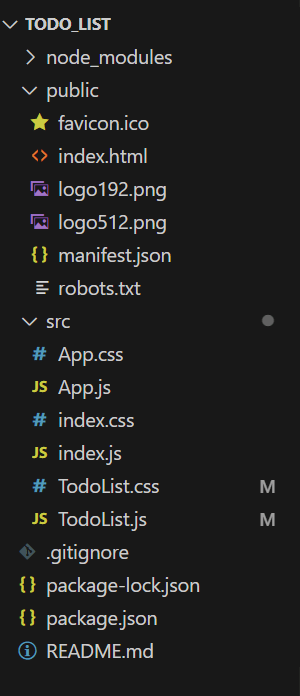
Here i am writing the code for index.html also so that it would be easy for the user to copy and paste the dependencies or cdn for bootstrap which we are using in our application
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<meta
name="description"
content="Web site created using create-react-app"
/>
<link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" />
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css"
integrity="sha384-9ndCyUaIbzAi2FUVXJi0CjmCapSmO7SnpJef0486qhLnuZ2cdeRhO02iuK6FUUVM"
crossorigin="anonymous"
/>
<script src="https://cdn.jsdelivr.net/npm/react/umd/react.production.min.js" crossorigin></script>
<script
src="https://cdn.jsdelivr.net/npm/react-dom/umd/react-dom.production.min.js"
crossorigin></script>
<script
src="https://cdn.jsdelivr.net/npm/react-bootstrap@next/dist/react-bootstrap.min.js"
crossorigin></script>
<!--
manifest.json provides metadata used when your web app is installed on a
user's mobile device or desktop. See https://developers.google.com/web/fundamentals/web-app-manifest/
-->
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
<!--
Notice the use of %PUBLIC_URL% in the tags above.
It will be replaced with the URL of the `public` folder during the build.
Only files inside the `public` folder can be referenced from the HTML.
Unlike "/favicon.ico" or "favicon.ico", "%PUBLIC_URL%/favicon.ico" will
work correctly both with client-side routing and a non-root public URL.
Learn how to configure a non-root public URL by running `npm run build`.
-->
<title>React App</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
</html>
Once the packages and dependencies are finished downloading, start up your IDE and locate your project folder. Letβs start Create our To do app . So here in the first step, we are going to target the App.js first
App.js
import './App.css';
import TodoList from './TodoList';
function App() {
return (
<>
<TodoList/>
</>
);
}
export default App;
Lets move to styling part so that we can style app.css
App.css
.container
{
width: 400px;
height: 400px;
background-color: rgb(255, 255, 255);
border-radius: 25px;
padding: 25px;
position: relative;
}
.container_model
{
width: 400px !important;
height: auto !important;
background-color: rgb(255, 255, 255);
position: relative !important;
}
.vertical-center {
margin: 0;
position: absolute;
top: 80%;
left: 50%;
-ms-transform: translate(-50%, -50%);
transform: translate(-50%, -50%);
}
.btn_area
{
border: none;
border-radius: 13px;
padding: 18px 138px;
font-weight: 600;
}
.space_betwwn
{
display: flex;
justify-content: space-between;
}
#dropdown-basic
{
background-color: #DDDDDD !important;
border: none !important;
}
.list-container {
max-height: calc(100vh - 570px); /* Adjust the height as needed based on your layout */
overflow-y: auto;
}
::-webkit-scrollbar {
width: 10px;
}
/* Track */
::-webkit-scrollbar-track {
background: #f1f1f100;
}
/* Handle */
::-webkit-scrollbar-thumb {
background: #c6c6c600;
border-radius: 5px;
}
/* Handle on hover */
::-webkit-scrollbar-thumb:hover {
background: #c6c6c600;
}
Lets move to Todo logic part where we have to write all the logic and our styling part . First we will start with TodoList.js
TodoList.js
Lets start to write TodoList.js code .
First we have to install bootstrap package because we are using bootstrap
Here is the npm package you can install to use some of its components.
npm install react-bootstrap bootstrap
Lets import some of code from bootstrap
And then after that we have to write all the code for the rest of the list . We have TodoList.js code for the todo List and after that TodoList.css .
import React from 'react'
import { useState } from 'react';
import Button from 'react-bootstrap/Button';
import Col from 'react-bootstrap/Col';
import Container from 'react-bootstrap/Container';
import Modal from 'react-bootstrap/Modal';
import Row from 'react-bootstrap/Row';
import Form from 'react-bootstrap/Form';
import Dropdown from 'react-bootstrap/Dropdown';
import './TodoList.css'
const TodoList = (props) => {
const [show, setShow] = useState(false);
const [activiy,setActivity] = useState("");
const [listData,setlistData] = useState([]);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
const addActivity = () =>{
setlistData(()=>{
const updatedList = [...listData,activiy]
console.log(updatedList)
setActivity('');
return updatedList
})
handleClose();
}
const removeActivity = (i) => {
const updatedListData = listData.filter((elem,id) => {
return i!=id;
})
setlistData(updatedListData);
}
const removeAll = () => {
setlistData([])
}
return (
<>
<div className='container'>
<div className='reset_container'>
<h2 className='contained-modal-title-vcenter2'>Todo List</h2>
<div className='icon'>
<svg onClick={removeAll} width="28" height="28" viewBox="0 0 28 28" fill="none" xmlns="http://www.w3.org/2000/svg">
<g clip-path="url(#clip0_19_209)">
<path d="M14 3.48809C16.7848 3.49746 19.4525 4.60903 21.42 6.57976L18.6504 9.34942H25.452C25.8066 9.34942 26.1467 9.20856 26.3974 8.95782C26.6482 8.70709 26.789 8.36702 26.789 8.01242V1.21076L23.8829 4.11692C21.9294 2.15429 19.437 0.81614 16.7219 0.272255C14.0067 -0.27163 11.1912 0.00326074 8.63253 1.06205C6.07386 2.12084 3.88736 3.91581 2.35044 6.21922C0.813516 8.52264 -0.00455399 11.2307 1.90684e-05 13.9998H3.48835C3.49144 11.2128 4.59991 8.54095 6.57056 6.5703C8.54121 4.59964 11.2131 3.49118 14 3.48809Z" fill="#949691"/>
<path d="M24.5116 14C24.5154 16.0793 23.9015 18.1128 22.7476 19.8425C21.5937 21.5722 19.9519 22.9201 18.0306 23.7151C16.1094 24.5101 13.9952 24.7164 11.9565 24.3078C9.91778 23.8991 8.04642 22.894 6.57994 21.42L9.3496 18.6503H2.3251C2.0297 18.6506 1.74649 18.7681 1.53761 18.977C1.32873 19.1859 1.21125 19.4691 1.21094 19.7645V26.789L4.1171 23.8828C6.07054 25.8455 8.56294 27.1836 11.2781 27.7275C13.9932 28.2714 16.8088 27.9965 19.3674 26.9377C21.9261 25.8789 24.1126 24.0839 25.6495 21.7805C27.1864 19.4771 28.0045 16.7691 27.9999 14H24.5116Z" fill="#949691"/>
</g>
<defs>
<clipPath id="clip0_19_209">
<rect width="28" height="28" fill="white"/>
</clipPath>
</defs>
</svg>
</div>
</div>
<div className="list-container">
{listData!=[] && listData.map((data,i) => {
return(
<>
<Form>
{['checkbox'].map((type) => (
<div key={`inline-${type}`} className="mb-3 space_betwwn">
<Form.Check
inline
label={data}
name="group1"
type={type}
id={`inline-${type}-1`}
/>
<Dropdown>
<Dropdown.Toggle variant="success" id="dropdown-basic">
</Dropdown.Toggle>
<Dropdown.Menu>
<Dropdown.Item onClick={()=>removeActivity(i)}>Remove</Dropdown.Item>
</Dropdown.Menu>
</Dropdown>
</div>
))}
</Form>
</>
)
})}
</div>
<div class="vertical-center">
<button className='btn_area' variant="primary" onClick={handleShow}>Add</button>
</div>
</div>
<Modal {...props}
aria-labelledby="contained-modal-title-vcenter"
centered show={show} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title id="contained-modal-title-vcenter">
Add Todo π
</Modal.Title>
</Modal.Header>
<Modal.Body>
<Container className='container_model'>
<Form>
<Form.Group className="mb-3" controlId="exampleForm.ControlTextarea1">
<Form.Label>Your thoughts π€</Form.Label>
<Form.Control as="textarea" value={activiy} onChange={(e)=>setActivity(e.target.value)} rows={3} />
</Form.Group>
</Form>
</Container>
</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button variant="primary" onClick={addActivity}>
Save Changes
</Button>
</Modal.Footer>
</Modal>
</>
)
}
export default TodoList
TodoList.css
Lets write our styling part for the todolist to make our tolist look like what we have mention in ui design .
.container {
display: flex;
flex-direction: column;
/* max-height: calc(100vh - 0px); */
}
.list-container {
margin-top: 20px;
flex: 1 1;
overflow-y: auto;
border-radius: 10px;
}
.btn-container {
margin-top: auto;
margin-bottom: 20px;
}
.reset_container
{
display: flex;justify-content: space-between;
}
#contained-modal-title-vcenter
{
font-weight: 700 !important;
}
.contained-modal-title-vcenter2
{
font-weight: 700 !important;
}
Let’s write our Index.css code. For the background change and the align the item at the center.
@import url('https://fonts.googleapis.com/css2?family=Inter:wght@100;200;300;400;500;600;700;800;900');
html,
body {
width: 100%;
height: 100%;
font-family: 'Inter', sans-serif;
display: flex;
align-items: center;
justify-content: center;
background: linear-gradient(90deg, #FFFBFB 0%, #FFF4F1 28.65%, #F7F9F1 56.77%, #F7FDF7 99.67%, rgba(255, 255, 255, 0.00) 100%);
}
Conclusion
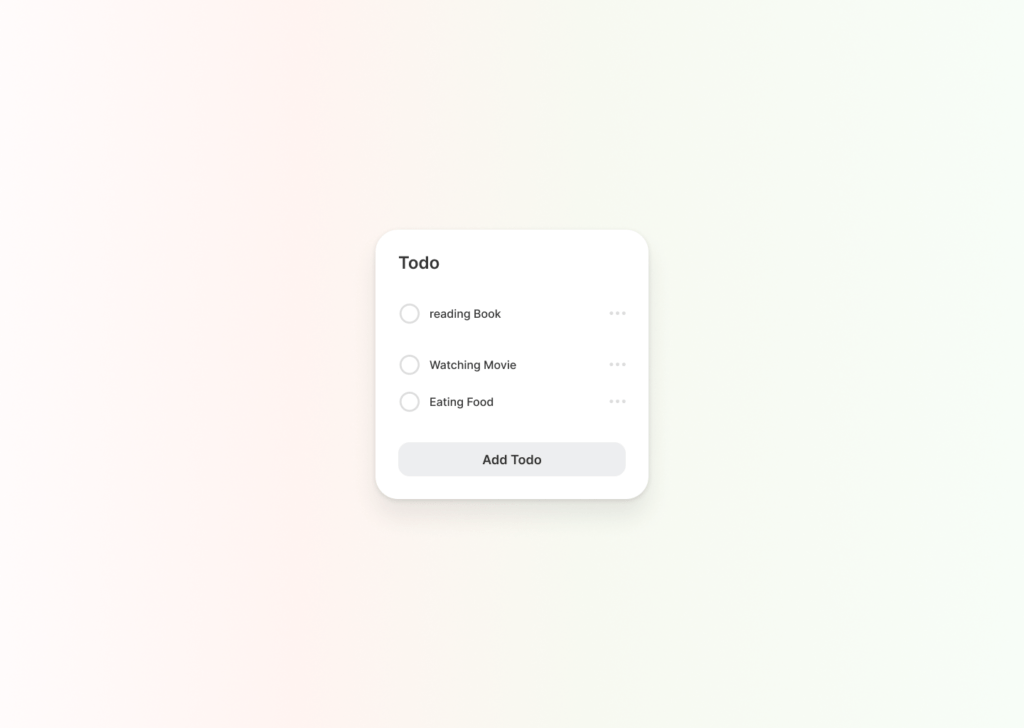
Hope you like this tutorial Any suggestions for any other application just comment and give your opinion. I hope you will successfully build this application and learn a lot from this application. Here is How to Build Todo App in React: Beginner Project we have built but in the upcoming days we will cover more beginner projects.
People are also reading:
- Crypto-currency website| Source Code Free Download
- How to Build A BMI Calculator in React JS β useState Hook & Conditionals
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers