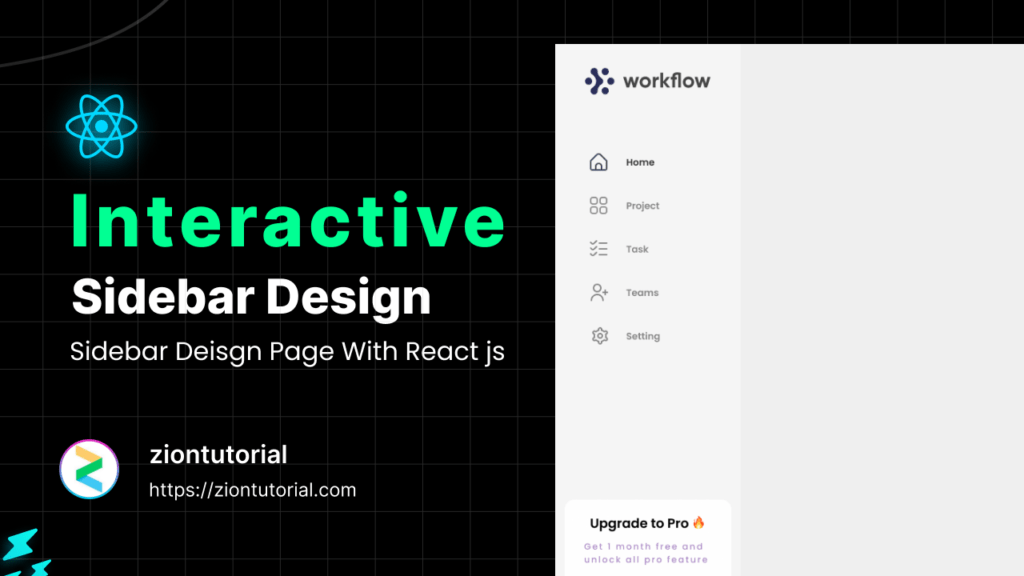
Hey, guys welcome to another blog How to make a sidebar in React JS | Dashboard Sidebar | Responsive Sidebar | Navbar React Router V6 where we will make sidebar designs from scratch. I hope you like the design If you face any problem during the code implementation of this sidebar you can comment in the comment box I will defiantly reply.
React is a JavaScript front-end library that may be used to build interactive user interfaces. Facebook created and keeps it up to date. It can be applied to the creation of mobile and single-page applications.
Demo of this Sidebar
Prerequisite:
- IDE of choice (this tutorial uses VS Code, you can download itΒ here for making a react sidebar)
- npm
- create-react-app
- react-router-dom
- useState React hooks
- sass
Basic Setup: To begin a new project using create-react-app, launch PowerShell or your IDE’s terminal and enter the following command:
The name of your project is “react-sidebar-dropdown,” but you can change it to something different like “my-first-react-website” for the time being.
npx create-react-app my-sidebar-app
Enter the following command in the terminal to navigate to your react-sidebar-dropdown folder:
cd my-sidebar-app
Required module: Install the dependencies required in this project by typing the given command in the terminal.
npm install react-router-dom
For icons, I have used flat icon interface icon
npm i @flaticon/flaticon-uicons
Link : Flaticon interface icon
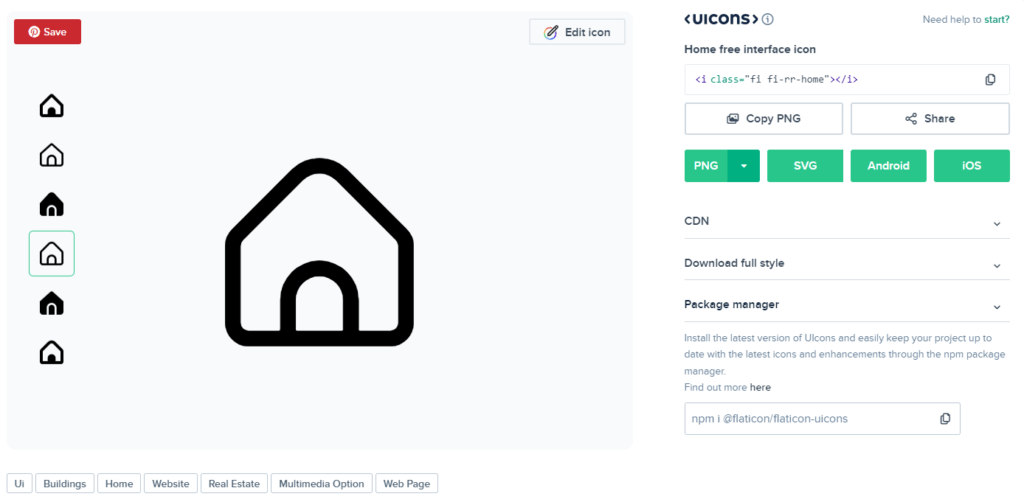
Folder structure
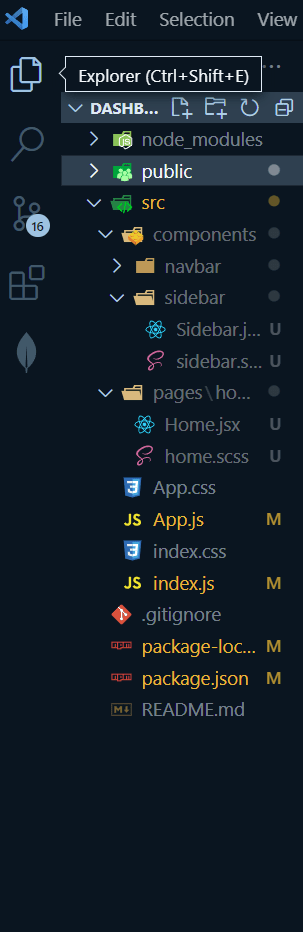
Once the packages and dependencies are finished downloading, start up your IDE and locate your project folder.
App.js
import { BrowserRouter, Routes, Route } from "react-router-dom";
import Home from "./pages/home/Home";
function App() {
return (
<div className="App">
<BrowserRouter>
<Routes path="/">
<Route index element={<Home />} />
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
Pages Folder structure
Home.jsx
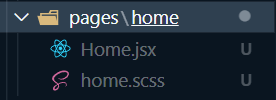
import React from "react";
import "./home.scss";
import Sidebar from "./../../components/sidebar/Sidebar";
// import Navbar from "./../../components/navbar/Navbar";
const Home = () => {
return (
<div className="home">
<Sidebar />
<div className="homeContainer"></div>
</div>
);
};
export default Home;
home.scss
.home{
display: flex;
.homeContainer{
flex: 6;
}
}
Lets jump to component folder where we have to design our sidebar using scss and folder structure of sidebar would be like this format.
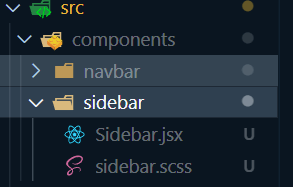
Lets first talk about sidebar .jsx file and the we will design our sidebar using scss.
Sidebar.jsx
import React from "react";
import "./sidebar.scss";
const Sidebar = () => {
return (
<div className="sidebar">
<div className="top">
<span className="logo">
<img src="Dark.png" alt="Katherine Johnson" />
</span>
</div>
<div className="center">
<ul>
<li>
<i className="fi fi-rr-house-blank icon"></i>
<span>Dashboard</span>
</li>
<li>
<i class="fi fi-rr-apps icon"></i>
<span>Project</span>
</li>
<li>
<i class="fi fi-rr-list-check icon"></i>
<span>Task</span>
</li>
<li>
<i class="fi fi-rr-users-alt icon"></i>
<span>Teams</span>
</li>
<li>
<i class="fi fi-rr-settings icon"></i>
<span>Setting</span>
</li>
</ul>
</div>
<div className="middle">
<div className="middle_card">
<h1 className="card_text">Upgrade to Pro π₯</h1>
<p>Get 1 month free and unlock all pro feature</p>
<button className="card_button">Upgrade</button>
</div>
</div>
<div className="center">
<ul>
<li>
<i class="fi fi-rr-interrogation icon"></i>
<span>Help</span>
</li>
<li>
<i class="fi fi-rr-exit icon"></i>
<span>Logout</span>
</li>
</ul>
</div>
</div>
);
};
export default Sidebar;
Then Let’s jump to our scss part where we will focus on the design of this sidebar.
sidebar.scss
.sidebar{
flex:1;
border-right: 0.5px solid rgb(230, 227, 227);
min-height: 100vh;
background-color: #F5F5F5;
.top{
height: 50px;
display: flex;
align-items: center;
justify-content: center;
padding: 40px;
.logo
{
font-size: 20px;
font-weight: bold;
color: #6439ff;
}
}
.center{
padding-left: 40px;
ul{
list-style: none;
margin: 0;
padding: 0;
li{
display: flex;
align-items: center;
margin-top: 30px;
padding: 5px;
cursor: pointer;
&:hover
{
background-color: #eaeaea;
border-radius: 0px 30px 30px 0px;
}
.icon{
font-size: 24px;
color: #868686;
}
span{
font-size: 13px;
font-weight: 600;
color: #888;
margin-left: 22px;
}
}
}
}
.middle
{
margin: 20px;
.middle_card
{
text-align: center;
background-color: white;
padding: 20px;
border-radius: 14px;
margin-top: 146px;
.card_text
{
display: flex;
align-items: center;
justify-content: center;
font-size: 16px;
}
p{
display: flex;
align-items: center;
justify-content: center;
color: #AA8AC3;
font-size: 12px;
padding: 10px;
}
.card_button
{
background-color:#BBD8F4 ;
border: none;
padding: 12px 63px;
border-radius: 34px;
font-size: 15px;
font-weight: 600;
}
}
}
}
Output

Conclusion
Hope you like this tutorial on How to make a sidebar in React JS | Dashboard Sidebar | Responsive Sidebar | Navbar React Router V6. For more such content please visit to the website.
People are also reading:
- Crypto-currency website| Source Code Free Download
- How to Build A BMI Calculator in React JS β useState Hook & Conditionals
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers