ReactJS Tutorial: How to Make a Responsive Navbar
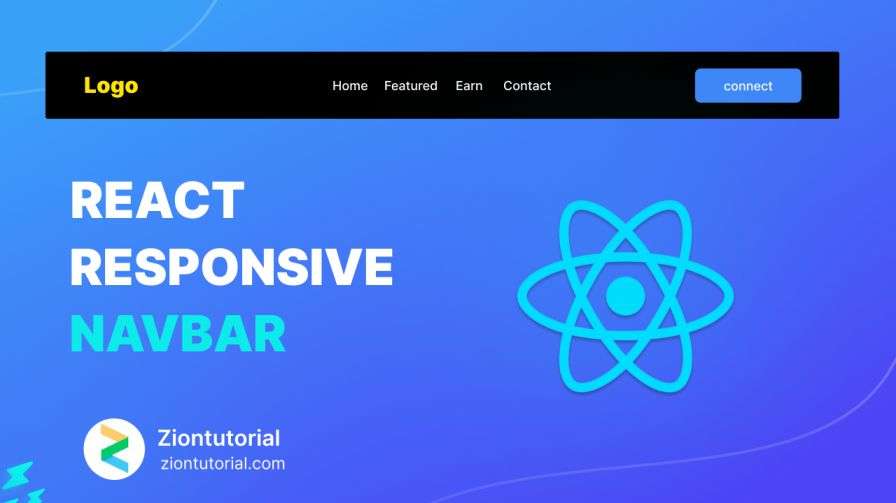
In this article, I will share how we can build a responsive Navbar using React js. That you can use in your project.
Create a navigation bar using reactJS and using to solve the following problem. which is how to create a responsive navigation bar using react js .
The modules Required are :
- npm
- create-react-app
To build this navigation bar you have to install a node in your local machine because we are downloading and using some of the packages from node modules.
After installing the node just check your node version by putting this command in your terminal
node -v
If you donβt please install the latest version.
All things are done you are ready to make a project using create-react-app so open your terminal and type :
npx create-react-app my-app
Now enter the following command into the terminal to access your navigation-bar folder:
cd my-app
I hope till this point you have successfully done the structure creation. Let’s move into coding and designing our responsive navbar using react js. Just follow all the steps you will Create a Responsive Navbar using ReactJS.
Index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
Index.
css
@import url('https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,100;0,200;0,300;0,400;0,500;0,600;0,700;0,800;0,900;1,100;1,200;1,300;1,400;1,500;1,600;1,700;1,800;1,900&display=swap');
:root {
--primary:#3F86F8;
--background:#f5f811;
}
.primary {
color: var(--primary);
}
*
{
margin: 0;
padding: 0;
box-sizing: border-box;
}
ul{
list-style-type: none;
}
a {
text-decoration: none;
color: #333;
}
.container {
max-width: 1240px;
margin: auto;
}
h1 {
font-size: 3.5rem;
line-height: 1.2;
}
h2 {
font-size: 2.4rem;
line-height: 1.2;
}
h5{
font-size: 1.1rem;
line-height: 1.2;
}
p{
font-size: 1.2rem;
}
.btn {
padding: 14px 32px;
border: 1px solid var(--primary);
background-color: var(--primary);
color: white;
border-radius: 10px ;
font-size: 1.1rem;
font-weight: 600;
cursor: pointer;
}
.btn:hover {
box-shadow:rgb(000/15%)0px 8px 24px;
}
input {
padding:12px 32px;
border: 1px solid var(--primary);
background: transparent;
border-radius: 24px 4px;
font-size: 1rem;
margin-right: .8rem;
font-family: 'Poppins',sans-serif;
}
@media screen and (max-width:768px) {
h1 {
font-size: 2.1rem;
line-height: 1.2;
}
h2 {
font-size: 1.5rem;
line-height: 1.2;
}
h5{
font-size: 1.1rem;
line-height: 1.2;
}
p{
font-size: 1rem;
}
.btn{
width: 100%;
margin: 1rem 0;
}
input {
width: 100%;
}
}
body {
margin: 0;
font-family: 'Poppins', sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
code {
font-family: source-code-pro, Menlo, Monaco, Consolas, 'Courier New',
monospace;
}
App.js
import React, {useState} from 'react'
import {FaBars, FaTimes} from 'react-icons/fa'
import './Navbar.css'
const Navbar = () => {
const [click, setClick] = useState(false)
const handleClick = () => setClick(!click)
return (
<div className='header'>
<div className='container'>
<h1 className='logo' >Logo</h1>
<ul className={click ? 'nav-menu active' : 'nav-menu'}>
<li>
<a href='/'>Home</a>
</li>
<li>
<a href='/'>Featured</a>
</li>
<li>
<a href='/'>Earn</a>
</li>
<li>
<a href='/'>Contact</a>
</li>
</ul>
<div className='btn-group'>
<button className='btn'>Connect Wallet</button>
</div>
<div className='hamburger' onClick={handleClick}>
{click ? (<FaTimes size={20} style={{color: '#333'}}/>) : (<FaBars size={20} style={{color: '#333'}} />)}
</div>
</div>
</div>
)
}
export default Navbar
Let’s work on the CSS part and which make our navbar looks awesome.
App.css
.header {
width: 100%;
height: 90px;
border-bottom: 1px solid #eee;
/* background: #fff; */
background: #000000;
position: sticky;
top: 0;
left: 0;
z-index: 10;
}
.header .container {
display: flex;
justify-content: space-between;
align-items: center;
height: 100%;
padding: 1rem;
}
.colors {
color: var(--primary);
}
.nav-menu {
display: flex;
}
.logo
{
color: #FFE600;
font-Size: 28px;
}
.nav-menu li {
padding: 0 1rem;
}
.nav-menu a {
font-size: 1rem;
font-weight: 600;
color: rgb(255, 255, 255);
}
.hamburger {
display: none;
cursor: pointer;
}
@media screen and (max-width:1240px) {
.hamburger {
display: flex;
}
.nav-menu {
position: absolute;
flex-direction: column;
justify-content: start;
top: 90px;
right: -100%;
width: 50%;
height: 100vh;
transition: 0.4s;
z-index: 2;
background: rgb(0, 0, 0);
border-left: 1px solid #eee;
}
.nav-menu.active {
right: 0;
}
.nav-menu li {
padding: 1rem;
width: 100%;
border-bottom: 1px solid #eee;
}
.nav-menu a {
font-size: 1.2rem;
}
}
Save all files and start the server by using the command.
Conclusion
We succeeded! We hope you like our guide on using ReactJS to How to Create a Responsive Navbar using ReactJS. Visit ziontutorial.com for similar projects and provide comments on which project I should cover next.
Check out this react and hooks-based BMI calculator. If you have any questions, comment. Please leave a remark on this project in the space provided. You can also join our Telegram channel, which is primarily accessible there, and ask any questions there.
Coding is fun!
People are also reading:
- Crypto-currency website| Source Code Free Download
- How to Build A BMI Calculator in React JS β useState Hook & Conditionals
- How To Create Sign Up Form In HTML and CSS
- Top Stunning Free Websites to Download Responsive HTML Templates 2021
- JavaScript Clock | CSS Neumorphism Working Analog Clock UI Design
- How to make Interactive Feedback Design Using HTML CSS & JS
- 5 amazing ways to earn money online as a side option .
- finest alternative of Chinese apps for Android and iOS
- Top Best 5 Fonts Of 2020 Used By Professional Graphic Designers
- React JS Mini-Project #2 Creating Simple Sum Calculator | Absolute beginners useState Hook & Conditionals